
The Fetch API is an activity for sending requests to the backend service endpoint on a website or application. The response from the API typically comes in JSON and XML data formats.
This data is then used to build applications on the front-end, making them more interactive and featuring dynamic data.
In the past, sending requests to the backend was done using Ajax or XML HTTP Request. However, now there is the Fetch API provided in the browser.
In this tutorial, we will learn:
How do we use the Fetch API in Alpine.js?
Ready?
Let's get started.
First, prepare the HTML file
Create an HTML file first, give it any name you like.
Then, inside it, we will use Alpine.js from the CDN.
For the CSS, we will use Bulma, so we don't have to write CSS code from scratch.
So, the HTML code will look like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
<title>Alpine JS Fetch API</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bulma@0.9.4/css/bulma.min.css" />
<script defer src="https://unpkg.com/alpinejs@3.x.x/dist/cdn.min.js"></script>
</head>
<body>
</body>
</html>
Prepare the API URL
For easier implementation, we will use a prepared Dummy API from JSON Placeholder.
This is an example of the JSON Placeholder endpoint URL.
https://jsonplaceholder.typicode.com/users
Next, let's try or test the API using Postman.
Postman is an application often used to test RESTful APIs. With Postman, we can modify methods to access the API.
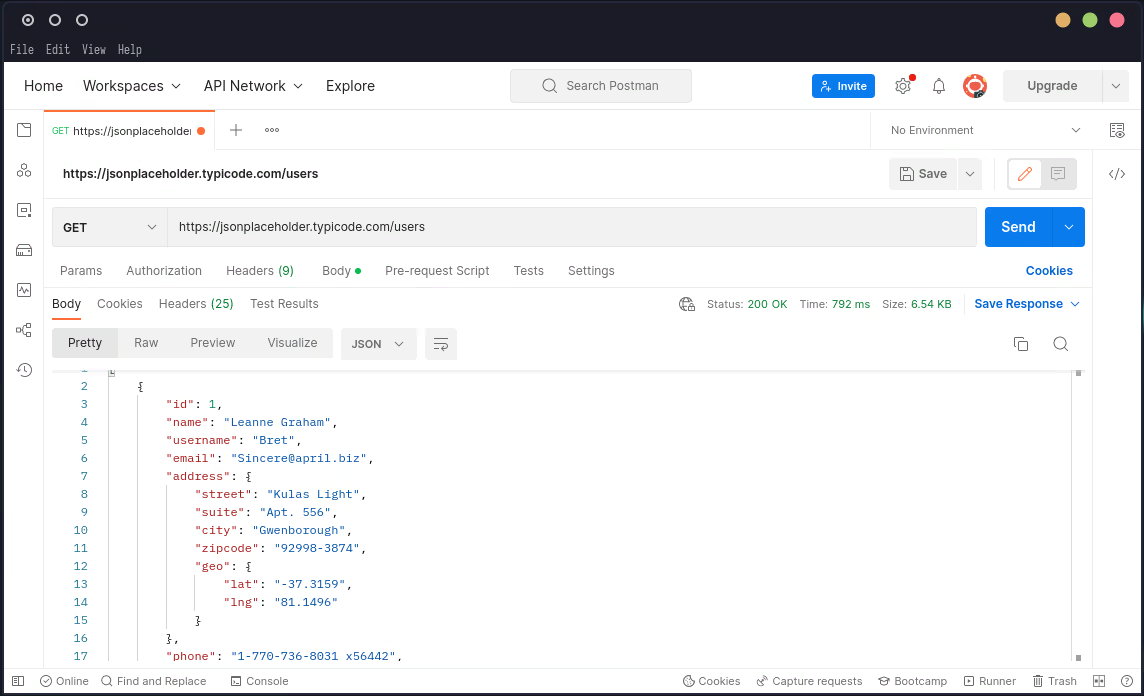
In this image, we are attempting an HTTP request to the /users
endpoint on JSON Placeholder.
Create a Table to Display Data
For a better presentation, we will use a table to display the data from the API since it consists of user data or identities.
First, create a table container:
Add code like this inside the <body>
tag.
<body class="container">
<table
x-data="{ users: [] }"
x-init="fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => users = data)"
class="table is-bordered bg-primary is-centered mt-6 mx-auto"
>
<!-- Place the table here -->
</table>
</body>
The intention of the code above is to:
- Store API data by declaring an empty variable, which is
users
- Retrieve or fetch an API in the form of a JSON file, then store it in the
users
variable, which is an empty array
Note: Ignore the value of the 'class' attribute for now, as it's a utility class from Bulma.
In Alpine.js, we create a new component with the x-data
attribute, and the purpose of x-init
is to execute a JavaScript function when the component is created.
In this case, we're creating a table component. Then, we call the fetch()
function when the table is displayed in the browser.
Next, we'll proceed to render the data to the table.
First, we create the table headers. For simplicity, match the headers with the "keys" present in the JSON object of the API. These keys can be observed using Postman.
Below is the code for the table headers.
<thead>
<tr>
<th class="has-background-link-light has-text-link">ID</th>
<th class="has-background-link-light has-text-link">Username</th>
<th class="has-background-link-light has-text-link">Name</th>
<th class="has-background-link-light has-text-link">Phone</th>
<th class="has-background-link-light has-text-link">Location</th>
<th class="has-background-link-light has-text-link">Company Name</th>
<th class="has-background-link-light has-text-link">Website</th>
</tr>
</thead>
After that, we will display user data by looping through each user object. Please add the following code:
<tbody>
<template x-for="user in users" :key="user.id">
<tr>
<td x-text="user.id" class="has-text-link-dark"></td>
<td x-text="user.username"></td>
<td x-text="user.name"></td>
<td x-text="user.phone"></td>
<td x-text="user.address.city"></td>
<td x-text="user.company.name"></td>
<td x-text="user.website"></td>
</tr>
</template>
</tbody>
In Alpine.js, the data that we want to loop through must be placed within the <template>
tag.
For further clarification, this <template>
tag serves as the location where we initialize the loop, specifically using the x-for attribute. This attribute retrieves data from the 'users' variable, with the :key
attribute determining how the data is looped based on the 'id' key.
Now, the complete HTML code will look like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
<title>Alpine JS Fetch API</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bulma@0.9.4/css/bulma.min.css" />
<script defer src="https://unpkg.com/alpinejs@3.x.x/dist/cdn.min.js"></script>
</head>
<body class="container">
<table
x-data="{ users: [] }"
x-init="fetch('https://jsonplaceholder.typicode.com/users')
.then(response => response.json())
.then(data => users = data)"
class="table is-bordered bg-primary is-centered mt-6 mx-auto"
>
<thead>
<tr>
<th class="has-background-link-light has-text-link">ID</th>
<th class="has-background-link-light has-text-link">Username</th>
<th class="has-background-link-light has-text-link">Name</th>
<th class="has-background-link-light has-text-link">Phone</th>
<th class="has-background-link-light has-text-link">Location</th>
<th class="has-background-link-light has-text-link">Company Name</th>
<th class="has-background-link-light has-text-link">Website</th>
</tr>
</thead>
<tbody>
<template x-for="user in users" :key="user.id">
<tr>
<td x-text="user.id" class="has-text-link-dark"></td>
<td x-text="user.username"></td>
<td x-text="user.name"></td>
<td x-text="user.phone"></td>
<td x-text="user.address.city"></td>
<td x-text="user.company.name"></td>
<td x-text="user.website"></td>
</tr>
</template>
</tbody>
</table>
</body>
</html>
For the results, you can open its HTML file in a browser.
The result will be like this:

What's Next?
Well, that's how you use the Fetch API in Alpine.js.
It's very easy, and moreover, it's only done within the HTML file.
Next, try to get creative by adding states like loading while performing the fetch API.
Hope this is helpful.